c#에서 사람들은 너무 무자비하게 Class만 사용한다. 하지만 실제 성능은 어떨까? stack과 heap의 차이라 너무 명백한 성능을 차이를 보이지만 똥인지 된장인지 찍어먹어봐야 아는 사람들이 있으니 이것을 보고 주니어를 졸업하도록 하자.
https://mdfarragher.medium.com/whats-faster-in-c-a-struct-or-a-class-99e4761a7b76
What Is Faster In C#: A Struct Or A Class?
What do you think is faster: filling an array with one million structs, or filling an array with one million classes?
mdfarragher.medium.com
위의 블로그를 참고하여 이 글을 작성하였다.
일단 테스트를 해보기 위해 프로그램을 아래와 같이 작성해보도록 하자.
public class BoxClass
{
public int Length { get; set; }
public int Width { get; set; }
public int Height { get; set; }
public BoxClass(int length, int width, int height)
{
Length = length;
Width = width;
Height = height;
}
}
public class BoxClassInheritance : BoxClass
{
public BoxClassInheritance(int l, int w, int h) : base(l, w, h)
{
}
//~BoxClassInheritance() { }
}
public struct BoxStruct
{
public int Length { get; set; }
public int Width { get; set; }
public int Height { get; set; }
public BoxStruct(int length, int width, int height)
{
Length = length;
Width = width;
Height = height;
}
}
class Program
{
public static bool TestA(int count)
{
var items = new BoxClass[count];
for (int i = 0; i < count; i++)
{
items[i] = new BoxClass(i, i, i);
}
return true;
}
public static bool TestB(int count)
{
var items = new BoxClassInheritance[count];
for (int i = 0; i < count; i++)
{
items[i] = new BoxClassInheritance(i, i, i);
}
return true;
}
public static bool TestC(int count)
{
var items = new BoxStruct[count];
for (int i = 0; i < count; i++)
{
items[i] = new BoxStruct(i, i, i);
}
return true;
}
static void Main(string[] args)
{
int iterations = 1000000;
System.Diagnostics.Stopwatch sw = new System.Diagnostics.Stopwatch();
sw.Start();
TestA(iterations);
sw.Stop();
Console.WriteLine($"Class => \t{sw.ElapsedMilliseconds} ms");
sw.Restart();
TestB(iterations);
sw.Stop();
Console.WriteLine($"Inheritance Class with destructor => \t{sw.ElapsedMilliseconds} ms");
sw.Restart();
TestC(iterations);
sw.Stop();
Console.WriteLine($"Struct => \t{sw.ElapsedMilliseconds} ms");
Console.ReadKey();
}
}
의도는 명확하다. Class와 Struct 중 무엇이 더 빠른가에 대한것이다.
실제로 코드를 돌려보면 아래와 같은 속도를 가진다.
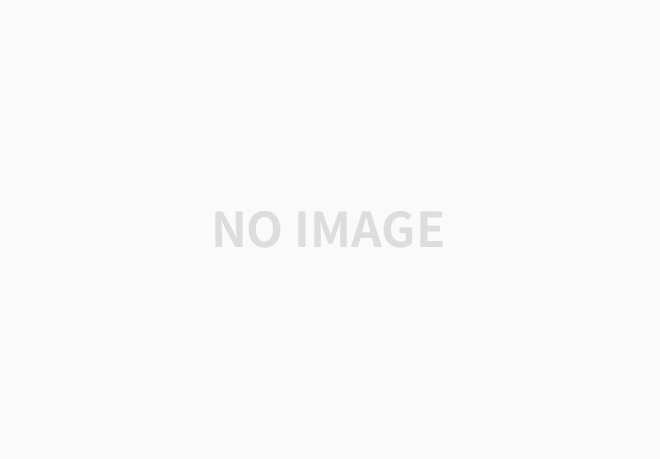
상속 받은 함수의 경우 무려 1.5배 차이가 나고 struct와 비교하면 더 심하게 벌어진다.
BoxClassInheritance에서 소멸자에 대한 주석을 해제하고 나면 더 심하게 벌어진다.
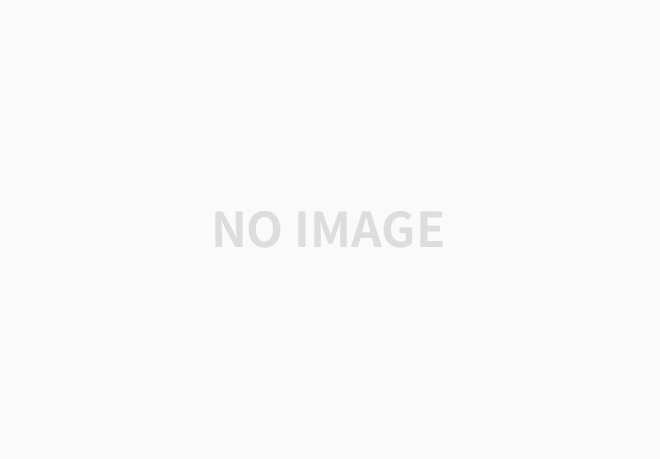
소멸자를 넣고다면 GC가 발동될때 소멸자를 타게됨으로 더 느리게된다.
여하튼 이러한 차이들은 데이터가 실제로 저장되고 운영되는 방식이 다르기 때문이다.
그렇다고 Struct가 빠르니 무조건 Struct만 사용해야 하나? 그렇게 생각할 수도있다.
그러면 안된다.
맨 위의 블로그에서는 다음과 같이 설명하였다.
- 3~40 byte 이상의 데이터를 저장하는 경우 Class 를 사용하라
- Reference Type을 저장할 때 Class를 사용하라
- 수천 개의 인스턴스를 저장하는 경우 Class를 사용하라
- 너의 List가 오래동안 유지되어야 할 경우 Class를 사용하라.
- 그외에 모든것들은 Struct를 사용하라
'프로그래밍 > C#' 카테고리의 다른 글
[C#] MessagePack-CSharp을 이용한 고성능 객체 직렬화 및 역직렬화 (0) | 2023.04.14 |
---|---|
[C#] List<object>를 특정 클래스 타입의 List로 캐스팅하는 방법 (0) | 2023.03.27 |
[C#] Oracle Bulk Insert (0) | 2022.04.01 |
[C#] Newtonsoft JsonConverter 사용하기 (1) | 2022.03.30 |
[C#] Oracle 연결 시 예외 발생 문제 (0) | 2022.03.11 |